Here you will find the most common Selenium WebDriver errors and the solutions to those errors. Enjoy 👍
Selenium C# errors
Element is not clickable at point (X, Y). Other element would receive the click…
Error Message
System.InvalidOperationException : unknown error: Element is not clickable at point (111, 700). Other element would receive the click: <div class=”et_social_heading”>…</div> (Session info: chrome=45.0.2454.93) (Driver info: chromedriver=2.15.322448 (52179c1b310fec1797c81ea9a20326839860b7d3),platform=Windows NT 6.1 SP1 x86_64)
The Problem
The problem here is that the element that you are trying to click is either covered by another element, or simply not visible. Here is an example. If you go to ultimateqa.wpmudev.host and try to click the Start Here button when you are a bit scrolled down, the header covers up the button. As a result, even though the button is on the page, it is not clickable because the header is covering it up.
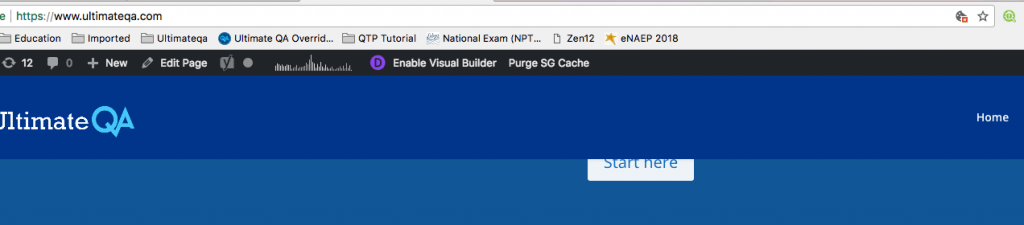
The Solution
To fix this problem, you need to make the element visible. Specifically, Selenium tries to click on the exact center of the element. Your options are to scroll up, or hover over an element to close it, or minimize an expanded element. Simply put, make sure that your element is visible for clicking. The center of the element that you want to interact with should not be obstructed by another element.
You can scroll the element into view like this:
Or you can use the actions class to move to element before interacting with it like this:
There is already an option for the browserVersion capability. Please use the instead.
Error Message
System.ArgumentException: ‘There is already an option for the browserVersion capability. Please use the instead.
Parameter name: capabilityName’
The Problem
With the introduction of the W3C spec, there will be changes to the DriverOptions.cs from Selenium. This means that the old way of setting the ChromeOptions or DesiredCapabilities will no longer be possible. So this will be a new way to make this happen.
The Solution
Set your DriverOptions through properties instead of passing in strings into the AddAdditionalCapability()
Here’s a code sample for C# and setting ChromeOptions:
Btw, if you’re struggling with Selenium and need my favorite resources, you might want to check these out.
Feel like your automation could be better?
Get a second pair of eyes on your automation.
Unable to copy file … chromedriver.exe
Error Message
Severity Code Description Project File Line Suppression State
Error Unable to copy file “C:\Users\User\Documents\Visual Studio 2015\Projects\UnitTestProject5\UnitTestProject5\chromedriver.exe” to “bin\Debug\chromedriver.exe
InnerException
The process cannot access the file ‘bin\Debug\chromedriver.exe’ because it is being used by another process
The Problem
The problem here is that your ChromeDriver is being used by another process. So when it’s trying to be copied, it can’t because it’s being used.
The Solution
Option A
- Open Task Manager and kill all chromedriver.exe processes
Option B
- Open Powershell command line.
- Execute command: Get-Process chromedriver | Stop-Process
- This will kill all processes at once
An exception with a null response was thrown sending an HTTP request… ConnectFailure …
Error Message
A exception with a null response was thrown sending an HTTP request to the remote WebDriver server for URL http://localhost:19409/session/7114d633a2adbc5105f3f6c7abc7c596/url. The status of the exception was ConnectFailure, and the message was: Unable to connect to the remote server
InnerException
Unable to connect to the remote server
The Problem
Compatibility of Selenium WebDriver with a browser is the culprit here. This is the largest burden in my behind, and has been for years now…
This will probably continue until Selenium WebDriver is a W3C standard that is integrated into each browser. Until then, we will keep having these issues.
This error occurred to me with:
- Chrome 55,56
- ChromeDriver 2.27
- Selenium WebDriver 3.0.1
The Solution
- Use ChromeDriver for all your test automation practice. Until I tell you it’s okay to use other Drivers.
- Make sure that your Chrome Browser and your ChromeDriver version are compatible.
- You can see this information on the ChromeDriver download page
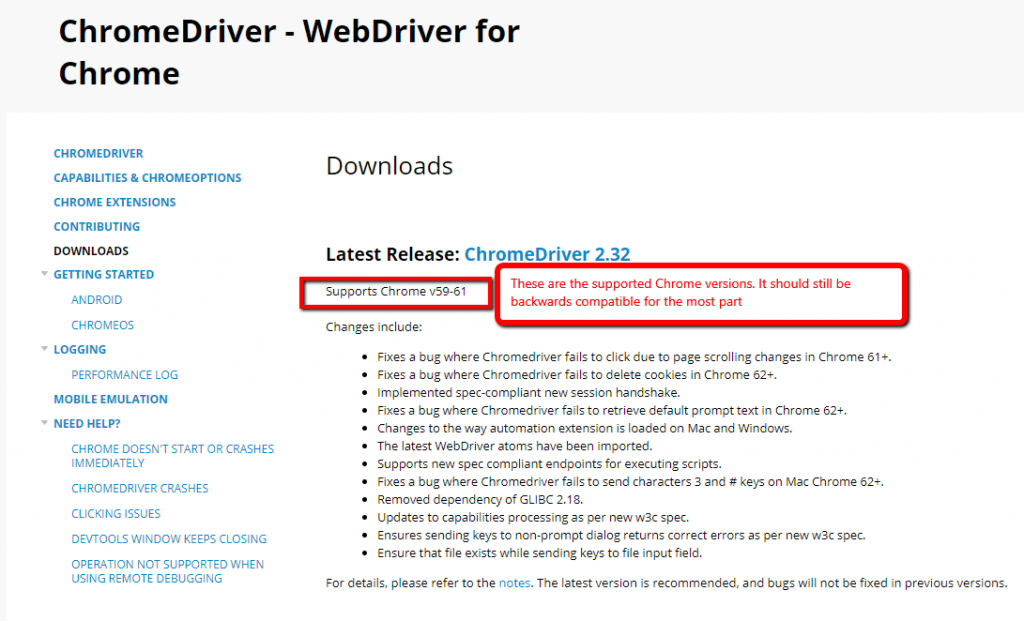
Upgrade your Chrome and your ChromeDriver if you must
- Warning: It’s almost impossible to downgrade ChromeDriver, so don’t try
Download the latest Selenium WebDriver API Nuget package Rebuild your whole solution with all of these new resources Try to run your tests again
- If this doesn’t work, restart your computer and try again.
An exception with a null response was thrown sending an HTTP request to the remote…
Error Message
Similar to the error above, but it’s slightly different in wording and message.
Selenium.WebDriverException: ‘A exception with a null response was thrown sending an HTTP request to the remote WebDriver server for URL http://localhost:25675/session. The status of the exception was ReceiveFailure, and the message was: The underlying connection was closed: An unexpected error occurred on a receive.’
InnerException
Unable to connect to the remote server
The Problem
You may have noticed that this page has one trend. Compatibility of Selenium WebDriver with a browser. This the largest burden in many of my behind, and has been for years now. This will probably continue until Selenium WebDriver is a W3C standard that is integrated into each browser. Until then, we will keep having these issues.
This error occurred to me with:
- Chrome 56
- ChromeDriver 2.27
- Selenium WebDriver 3.0.1.0
The Solution
- Update your Chrome browser to version 57.0
- This did the trick for me. Keep in mind that maybe your versions of browser and ChromeDriver may be different. However, if you receive this error, just upgrade your browser until it fits into the support matrix of ChromeDriver release logs.
System.TypeInitializationException
System.TypeInitializationException: System.TypeInitializationException: The type initializer for ‘Framework.Browser’ threw an exception. —> OpenQA.Selenium.WebDriverException: Failed to start up socket within 45000 ms. Attempted to connect to the following addresses: 127.0.0.1:7055.
Or something like this?
OpenQA.Selenium.WebDriverException : Unexpected error. System.Net.WebException: Unable to connect to the remote server —> System.Net.Sockets.SocketException: No connection could be made because the target machine actively refused it
Today is your lucky day because I am going to help you resolve these really annoying errors!
The Problem
The problem is a standard compatibility issue between Selenium WebDriver and your version of a browser. As I am writing about this, the latest WebDriver version is 2.53.1. The latest Chrome version is 51.0 and the latest Firefox version is 46.0. Therefore, the Selenium WebDriver API does not get updated as often as Chrome or Firefox.
When different types of browsers such as Chrome or Firefox get updated, there is a chance that this update will break the Selenium API.
In our industry, this is known as a regression. Therefore, when you get the latest version of your browser, you may be using a Selenium WebDriver version that does not support the latest features of that browser. At this point, you will receive an ugly error that looks like this:
System.TypeInitializationException
Now, you will spend the next day wondering how your Selenium code used to work yesterday and today it just stopped working.
The Solution
Downgrade your appropriate browser to the version supported by the latest Selenium Driver. This might be Firefox, ChromeDriver, IEDriver and so on.
In this example I teach you how to downgrade Firefox.
System.InvalidOperationException : unknown error: Chrome version must be…
Error Message
System.InvalidOperationException : unknown error: Chrome version must be >= 54.0.2840.0
The Problem
Another issue with compatibility of ChromeDriver with Chrome Browser. In this case it was Chrome version 48.0 not working with ChromeDriver version 2.27
The Solution
Upgrade your Chrome browser to the version supported by ChromeDriver. For example, ChromeDriver 2.27 supports Chrome 54 – 56 according to their release notes.
Could not copy “C… chromedriver.exe” to {some path}..
Error message
Could not copy file “C:\…chromedriver.exe” to “…\chromedriver.exe
Solution
See this error above “Unable to copy file … chromedriver.exe”. It’s the same exact problem
Unhandled Exception: System.IO.FileNotFoundException: Could not load file or assembly… for a driver
The solution
Is here
ExpectedConditions’ is obsolete: ‘ implementation… deprecated
Error message
ExpectedConditions’ is obsolete: ‘The ExpectedConditions implementation in the .NET bindings is deprecated and will be removed in a future release. This portion of the code has been migrated to the DotNetSeleniumExtras repository on GitHub (https://github.com/DotNetSeleniumTools/DotNetSeleniumExtras)’
The problem
Winter is coming! And so is Selenium 4.0 🙂 That’s exciting news because it means that it’s getting better. However, this also means that there are some things that will be deprecated. ExpectedConditions.cs will be moving to a different Nuget package. Right now, if you are using Selenium 3.X, this is just a warning. Later, you will not be able to do this.
The Solution
I outlined the solution in depth here
NoSuchElementException: no such element: Unable to locate element
Error message
OpenQA.Selenium.NoSuchElementException: no such element: Unable to locate element: {“method”:”partial link text”,”selector”:”Master Automation With Selenium”}
(Session info: chrome=81.0.4044.138)
(Driver info: chromedriver=2.29.461591 (62ebf098771772160f391d75e589dc567915b233),platform=Windows NT 10.0.18362 x86_64)
The problem
The element cannot be found on the page. This can happen when you are using an incorrect locator for your element, or when the element has not been yet loaded on the page.
The Solution
This problem has two possible solutions:
Solution A
If the problem is that the locator is incorrect, try using a different locator (by Id, Name, Xpath etc) and make sure that the locator corresponds to a single element on the page.
Solution B
If the problem is related to the loading time, add a wait, so Selenium will wait a few seconds before throwing the exception if the element is not found.
- Implicit wait (not really recommended)
WebDriverWait wait = new WebDriverWait(Driver, TimeSpan.FromSeconds(5));
Or
Driver.Manage().Timeouts().ImplicitWait = TimeSpan.FromSeconds(5);
- Explicit wait
First, download the DotNetSeleniumExtras.WaitHelpers Nuget package.
Add this using statement to your class:
using ExpectedConditions = SeleniumExtras.WaitHelpers.ExpectedConditions;
Then add the explicit wait to your method:
WebDriverWait _wait = new WebDriverWait(Driver, TimeSpan.FromSeconds(5)); _wait.Until(ExpectedConditions.ElementIsVisible(locator));
You can find more information on using the Explicit wait here.
Selenium Java Errors
Error:java: error: release version 5 not supported
java.lang.IllegalStateException: The driver is not executable: …/chromedriver
Error Message
java.lang.IllegalStateException: The driver is not executable: /Users/nikolayadvolodkin/Documents/source/java/selenium-java/resources/mac/chromedrive
The Problem
The issue is that you need to download the correct Chromedriver version for your OS
The Solution
The solution is here, see my comment in SO as well.
“chromedriver” cannot be opened because the developer cannot be verified.
Error Message
“chromedriver” cannot be opened because the developer cannot be verified.
The Problem
Mac OS security stuff…
The Solution
The solution is on SO. Either answer 1 or 2 will fix this
The driver executable does not exist
Error Message
“The driver executable does not exist”.
The Problem
(1) The path of the file may not be correct or (2) chrome driver may not be compatible with your chrome browser.
The Solution
- Copy into your local file the following paths:
Path for Mac:
System.setProperty("webdriver.chrome.driver", "resources/mac/chromedriver")
Path for Windows:
System.setProperty("webdriver.chrome.driver", "resources/windows/chromedriver.exe");
2. Make sure that you download the correct version of chromedriver to your machine depending on your OS: Download here.
Selenium Java Errors – Maven errors
[ERROR] Source option X is no longer supported. Use Y or later.
Error Message
For this we will use for example X being version 1.5 and Y being version 1.6:
[ERROR] Source option 1.5 is no longer supported. Use 1.6 or later.
[ERROR] Target option 1.5 is no longer supported. Use 1.6 or later.
The Solution
Need to set your maven.compiler.source to at least 1.6
<properties>
<maven.compiler.source>1.6</maven.compiler.source>
<maven.compiler.target>1.6</maven.compiler.target>
</properties>
Solution 2
You might need to add the Maven compiler plugin to your POM.xml. Make sure that you set the correct source and target based on your JDK.
Btw, if you’re struggling with Selenium and need my favorite resources, you might want to check these out.
Cannot start compilation: the output path is not specified for module “xyz” Specify the output path in Configure Project.
Error Message
Cannot start compilation: the output path is not specified for module "xyz" Specify the output path in Configure Project.
The Problem
The issue is that you need to set an output path in your Intelli J.
The Solution
I was able to resolve this problem by setting a path in the Project compiler output. I simply create an `out` folder in my parent directory and specified the path to point to this directory.
Error Message
org.openqa.selenium.SessionNotCreatedException: Could not create a session: You must enable the ‘Allow Remote Automation’ option in Safari’s Develop menu to control Safari via WebDriver.
Build info: version: ‘3.141.59’, revision: ‘e82be7d358’, time: ‘2018-11-14T08:17:03’
Driver info: driver.version: SafariDriver
The Solution
We need to enable Allow Remote Automation in Safari like this
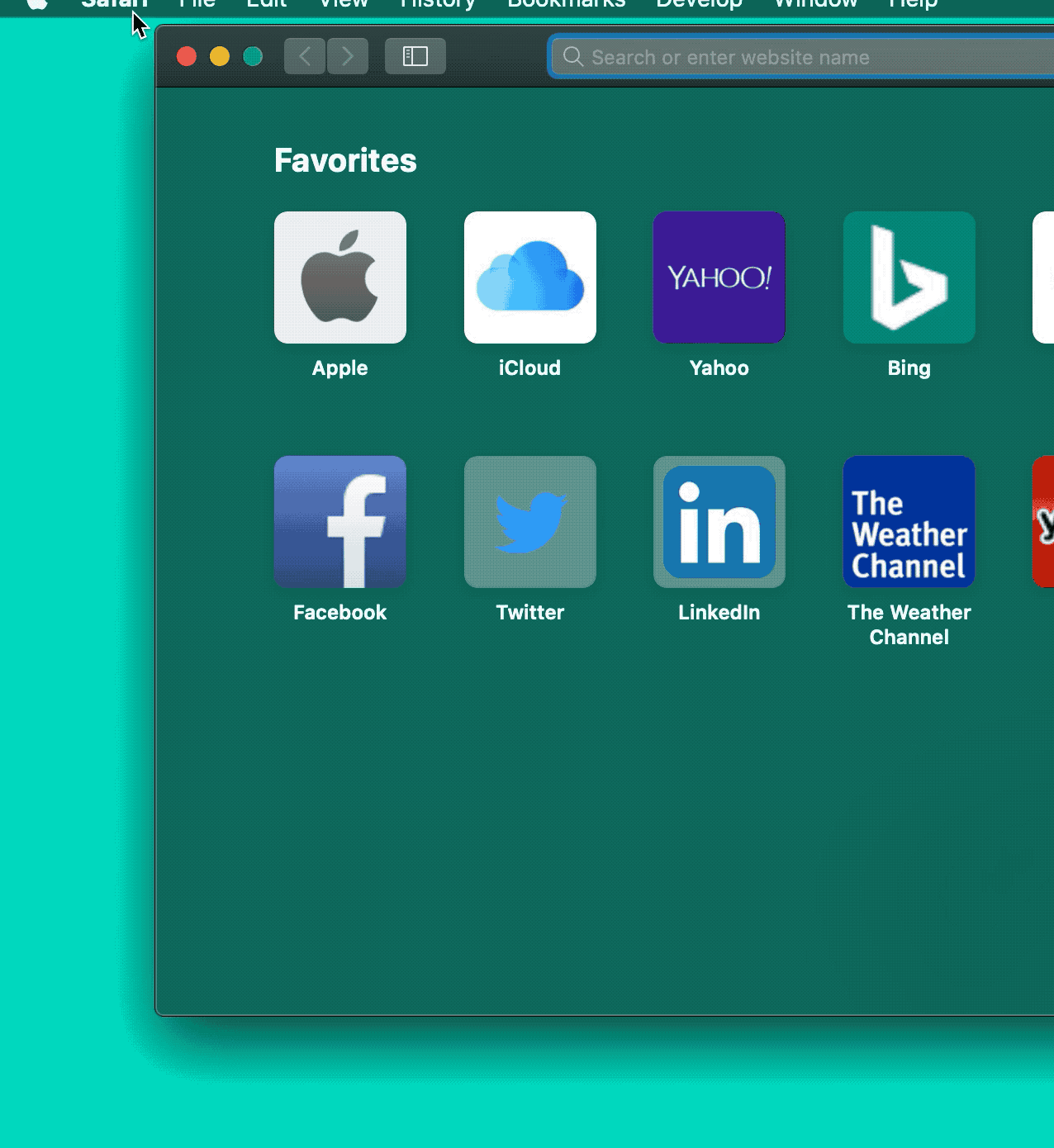
Visual Studio Errors
If you are using Visual Studio with C# and MsTest for your Selenium automation, you can find common Visual Studio error here.
Miscellaneous Errors
Mac/Linux is not returning environment variable values from terminal
Error Message
When trying to read environment variables from your test code like this
protected static final String SAUCE_USERNAME = System.getenv("SAUCE_USERNAME");
Or even trying to read it in terminal like this
echo $SAUCE_USERNAME
the string comes back as empty.
The Solution
If your variables are stored in ~/.bash_profile then you need to make sure that your default terminal shell is bash and not something else.
Follow these steps:
//1. check your default shell in a terminal echo $0
if it doesn’t say bash then we need to change it
//2. get the path of your bash type -a bash // bash is /bin/bash
then change to bash using the location from above
chsh -s /bin/bash
close and then reopen the terminal and rerun your commands to access the variables.
Summary
Hope this was helpful?
Is there an error that you need help resolving? Please comment below and I’ll try to resolve it for you.
I got this exception while executing a simple program to test selenium:
Unhandled Exception: System.TypeLoadException: Could not load type ‘OpenQA.Selenium.Chrome.ChromeDriver’ from assembly ‘WebDriver
What is the problem?
Are you using Java bindings? If so, I am not familiar with Java binding errors.
OpenQA.Selenium.WebDriverException : A exception with a null response was thrown sending an HTTP request to the remote WebDriver server for URL http://hub-cloud.browserstack.com/wd/hub/session/6a9462564325d212d46b32980d2f14252f1e8313/keys. The status of the exception was KeepAliveFailure, and the message was: The underlying connection was closed: A connection that was expected to be kept alive was closed by the server.
—-> System.Net.WebException : The
Problem occurs when tests run using TFS release nightly build
I am trying to create POM Framework. Code was working till yesterday and from today same code is giving “ArgumentException was unhandled by user code”
An exception of type ‘System.ArgumentException’ occurred in WebDriver.Support.dll but was not handled in user code
Additional information: The SearchContext of the locator object cannot be null
I have sanity script – which is calling test script and that script is refering to page object class. Error is coming in the pageobject class @ PageFactory.InitElemtns(driver, this); line below:
public LandingPage(IWebDriver driver)
{
this.driver = driver;
PageFactory.InitElements(driver, this);
}
DOn’t use PageFactory it has tons of issues. That’s probably why you are getting that error. Use properties to just return your elements.
If you want to know how to structure you page objects well, take a look at this Page Objects Course
wise advice
Hi Nikolay, I am loving the course!
I have encountered some strange issues in Visual Studio. I am using Windows 10 Home, Visual Studio Professional 2017, ChromeDriver 2.33, supports Chrome version 62+, using Chrome Version 62.0.3202.75 and Selenium.Support and Selenium.Webdriver version 3.7.
1. In “How to download and install Selenium Webdriver” I have noticed as you type “var driver = new ChromeDriver();” the “using OpenQA.Selenium.Chrome;” gets added automatically, not in my case. I had to type in myself.
2. Intellisense is not working.
3. Chromedriver > Properties (“How to download Chrome Driver) is missing “Advance” category hence “Copy to output directory” is unavailable.
What am I missing?
Thanks in advance.
Excellent 🙂
Hi Nikolay, can you please answer 3rd. question, i.e. In Visual Studio > Solution Explorer, Chromedriver > Properties (“How to download Chrome Driver” video) is missing “Advance” category hence “Copy to output directory” is unavailable.
What can I do to fix this?
Thanks.
Just wanted to mention that I found the solution to Visual Studio 2017 Intellisense fix. Apparently there is a bug!
Hello,
I am having trouble identifying an element. My application has a left navigation menu called New and when expanded it shows sub menus.
I was able to identify New and then clicked on it. But failing to identify the sub menu.
This is a part of the code that I have so far …
IWebElement navmenu = driver.FindElement(By.XPath(“//*[@id=’subMenuModules’][8]”));
navmenu.Click(); //success
Thread.Sleep(TimeSpan.FromSeconds(15));
IWebElement contactclick = driver.FindElement(By.XPath(“//*[@id=’collapse’]/paper-menu/div/paper-item[1]”));
contactclick.Click(); //failing .
https://uploads.disquscdn.com/images/dd5530de7ce43e4a7301f4f35bdaff017ab30c90da6ff9a4c9c779bff83c96e1.png
Please see attached screenshot for the HTML.
Thank you and appreciate your support.
-Sharmila
Do you need to hover over an element to click it? Usually that is the case. Especially this looks like a submenu. So you probably need to hover on an element. Or click some element first. THen open the menu, then proceed?
Hi, I have problems with IE 11 – (IE11 in test requirements)
What I did:
I have downloaded IE driver from http://selenium-release.storage.googleapis.com/index.html?path=3.9/
name of the file IEDriverServer_Win32_3.9.0.zip -> unzipped -> Placed to WebBrowserFactory =
I can run IE11 browser and open target website
What is wrong:
If I run 6 tests together
I can’t open any locator – So FIRST test is ok – but for rest five tests I have error bellow:
————–
“OpenQA.Selenium.NoSuchElementException: Unable to find element with css selector == #loginUsername”
————-
but same code works perfect in Google Chrome
Latest version try WebDriver/IEDriver v3.9, v3.9.1, v3.8 – different version combinations
What do you mean run tests “together”. Do you mean in parallel, all at the same time? Or do you mean as a suite of 6 tests, one at a time?
If it’s the former. The problem isn’t I É. The problem is that your tests are probably not independent, making parallel execution impossible.
My bad. Not good explanation. So I have 6 tests now. not in parallel – And I click Run All at Test explorer
Okay, so then it might be a problem with IE then. Remember that different browsers render HTML differently. In fact, I feel like IE doesn’t support CSS locators if I remember correctly?
May I as why you are using IE? Almost nobody in the world uses that browser. In fact, Microsoft no longer suports it – https://venturebeat.com/2016/01/12/microsoft-ends-support-for-ie8-ie9-ie10-and-windows-8/
That’s why they created Edge.
So although I might be able to help you fix the running tests on IE. I think that we’re placing a band aid on a larger problem that will come back and kill your automation. The problem being that you use IE. At worst, use Edge. At least it’s build with Selenium integration.
Sorry if that’s not the answer you’re looking for, I need to be honest and help people to come up with the best solution possible.
May be…. I use these
private IWebElement UserField => Driver.FindElement(By.Id(“loginUsername”));
private IWebElement PasswordField => Driver.FindElement(By.Id(“loginPassword”));
private IWebElement LoginButton => Driver.FindElement(By.Id(“loginSignInButton”));
That’s not what your error shows:
“OpenQA.Selenium.NoSuchElementException: Unable to find element with css selector == #loginUsername”
Also, the question is, do these tests work in Chrome fine? But then don’t work in IE? If the answer is yes, it’s your locators. You need a different strategy for IE.
I am going through the examples in the LightPomFrameworkTutorial package. I am getting a build error on many of the examples. The error is “AutomationResources.dll could not be found”
As a specific example, I am working with SampleApp2. When I build the solution I am getting the following error:
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘D:VS ProjectsLightPomFrameworkTutorialAutomationResourcesbinDebugAutomationResources.dll’ could not be found SampleApp2 D:VS ProjectsLightPomFrameworkTutorialSampleApp2CSC 1 Active
I looked in the ‘AutomationResourcesbinDebug’ folder and as the error states, there is no AutomationResources.dll there.
This same error is coming up during building of many of the examples.
How can I correct this error?
Thanks,
Jim
do you have chromedriver.exe being ran in the background? If so, kill it and try again
Wow, that worked. You’re amazing!!!
A number of the examples in “ElementIdentification.cs” (that show the various ways to use an Element Locater) are failing.
The following is one example of the errors I am getting:
ProjectsLightPomFrameworkTutorialElementInteractionsElementIdentification.cs:line 44
Result Message:
Test method ElementInteractions.ElementIdentification.ElementIdentificationTest threw exception:
OpenQA.Selenium.NoSuchElementException: no such element: Unable to locate element: {“method”:”name”,”selector”:”NameExample”}
(Session info: chrome=65.0.3325.181)
Can you use this url to test against instead? https://web.archive.org/web/20170808214449/http://www.qtptutorial.net:80/automation-practice/
My new page is not fully finished
Sent from TypeApp
That doesn’t work (I already tried it). That link is the link I sent you from the web archives (WaybackMachine).
The problem is, even though the web pages are archived, the contents remain the same.
For example, if you look at the underlying code for the “Click This Button Using ‘Name’ Property”, you will see …
which is still pointing to qtptutorial.net.
Hey Jim,
I’ll need to finish adding all of the elements here https://ultimateqa.wpmudev.host/simple-html-elements-for-automation/ . Please give me a day or 2 to finish that.
No problem. I’ll move on to ‘Implicit and explicit waits’ in the mean time. Thanks for all your efforts!
Hi Nikolay, great course, really enjoying it so far!
I’m just having a few issues with nlog and getting data written to a file in the following location: c:temp${appName}Debug.log”
I’ve tried copying the code from the config file on your github repository but have had no joy. However, when I debug the tests and hit the line of code relating to the logging of data I get an error as below. The test then runs successfully but I don’t see a log file being generated(screenshot 1)
https://uploads.disquscdn.com/images/b3f65c9ef623284e06d5b8c6197c6092e9b62248a892203ea421d1f1ab479e6b.png
For reference the HomePage code is below too(screenshot2)
I can recommend that you try 2 things:
1. Compare your Nlog.config to mine. And also your source code to mine. What’s the difference?
2. Try to start Visual Studio as administrator and let me know if that helps.
Sorry Nikolay, here is the second screenshot
https://uploads.disquscdn.com/images/85ffeba4da5f9167c223b20032a372f8139eccd3006ddafe0c1d557698e66ffe.png
Hi Nikolay,
I found an issue in one of the videos I thought you’d want to be aware of.
Answer to why the test is flaky:
The audio cuts out around 0:41
Besides that, this is the most amazing Selenium (and C#) training I have ever seen!
Thank you for putting this course together.
Jim
Hey Jim, thank you so much. I’ll have that fixed this weekend! Appreciate you taking time out of your day to report this issue 🙂
Thanks for the compliment as well. I’m actually updating a bunch of stuff, so it’s about to be even better 🙂
Hey Jim, that video is fixed!
Awesome, thank you!
Hi Nikolay,
Just fyi, the section:
Different types of Visual Studio projects > Class library project
doesn’t have audio until 3:34
Thanks sir, it’s fixed
In the code for the lesson on Element Identification, the following code needs to be changed for this test to pass:
From inside ElementIdentification.cs …
From:
[TestMethod]
[TestCategory(“Element Interrogation”)]
public void ElementInterrogation()
{
driver.Navigate().GoToUrl(“https://ultimateqa.wpmudev.host/automation/”);
var myElement = driver.FindElement(By.XPath(“//*[@href=’http://courses.ultimateqa.com/users/sign_in’]”));
}
To:
[TestMethod]
[TestCategory(“Element Interrogation”)]
public void ElementInterrogation()
{
driver.Navigate().GoToUrl(“https://ultimateqa.wpmudev.host/automation/”);
var myElement = driver.FindElement(By.XPath(“//*[@href=’https://courses.ultimateqa.com/users/sign_in’]”));
}
So, the change is from @href=’http: to @href=’https:
I hope this helps,
Jim
Thanks Jim 🙂
All fixed. Yea, we updated to HTTPS and didn’t update the code.
In C#, does anyone know how to check to see if a connection (driver) is already connected?
I am writing automation for a device and want to check for the existence of some elements on the html page but I only want to do it if there is already a web driver connected to the web page.
I want to do something like this:
if Driver is connected
run this test
else
send a message to user that a web driver must already be active (connected).
Thanks,
Jim
Hey Jim, what are you trying to accomplish?
I am writing automated tests for a device and I have a test which verifies that certain header and footer elements are on every page. I would like to run this test without having to call the login section (which connects to the page and logs in a user).
Hence, I am trying to find a way to check and see if there is a ‘driver’ instance running (which connects this page … the html page is showing in the browser).
If the html page is running in a browser, I want to run this test to verify that the elements are present but if the html page is not running in the browser (hence it is not connected and a webdriver is not running) I want to NOT run the script.
Does this make sense? In summary, I am trying to find a way to check if the html web page I am testing is running, if so, run this test, if not don’t run the test.
Maybe this can’t be done, I don’t know. I haven’t been able to find a way.
Thanks,
Jim
If you have requirements that must be checked in a single test, then use that test to check the requirements. You shouldn’t be using 2 tests to validate the requirements of one test. One test, one driver. All tests must be atomic and isolated. Hope this helps.
Thanks Nikolay, I appreciate it.
Hey Nikolay,
I am taking part in your udemy course ‘Selenium WebDriver-Working With Elements’. I am currently watching video no 58 and I have a problem with opening Network Tab in Firefox. Firefox is opening and google page is opening, but then nothing happens. I have Firefox 61. Here’s my code:
var _driver = new FirefoxDriver();
var _actions = new Actions(_driver);
_driver.Navigate().GoToUrl(“https://www.google.com/”);
_actions.KeyDown(Keys.Control).KeyDown(Keys.Shift).SendKeys(“e”).Perform();
_actions.KeyUp(Keys.Control).KeyUp(Keys.Shift).Perform();
Ania
Ania
Hey Nikolay,
I am taking part in your udemy course ‘Selenium WebDriver-Working With Elements’. I am currently watching video no 58 and I have a problem with opening Network Tab in Firefox. Firefox is opening and google page is opening, but then nothing happens. I have Firefox 61. Here’s my code:
var _driver = new FirefoxDriver();
var _actions = new Actions(_driver);
_driver.Navigate().GoToUrl(“https://www.google.com/”);
_actions.KeyDown(Keys.Control).KeyDown(Keys.Shift).SendKeys(“e”).Perform();
_actions.KeyUp(Keys.Control).KeyUp(Keys.Shift).Perform();
Thanks,
Ania
I was wondering if anyone would help me out here. I’ve learned a LOT from this class but one thing I seem to be missing (I can’t find the answer anywhere) is how can I replace the “Assert” commands with something like a “Verify” or a Soft Assert within C#.
When I have an assert that unexpectedly fails, I get an exception which stops the tests. What I am trying to figure out is what can I use in C# and Selenium such that when I get a failure of any kind, the failure is reported and the tests continue to the end.
I am automating some functional tests (rather than unit testing) and, although maybe unconventional, I have tests that test multiple items on an html page (I have no choice, I was instructed to do it this way). I want my entire test sequence (which tests multiple buttons, check boxes, pull down menus) that all have asserts to be completed. If one assert fails, I need that failure to be reported somewhere (I haven’t figured out how to get my test results to output to an HTML file) and then continue the test until the end.
So, how can I run a test sequence with multiple asserts (again, I have no choice) and if one of the asserts fails, the entire test sequence continues until the test sequence has completed.
I need an output report that lists all the passes and failures and NOT have the test stop until it has completed.
Thanks,
Jim
There is no such concept because it’s not a valid concept in test automation. There’s a saying, fail fast and fail early so that you get real value from your test. If you don’t want to Assert and fail, then just don’t assert. If you just want to report, then just use the Reporter class. Assertions are for stopping the test. Reporter is for reporting steps. Check out the Reporting section of the course, it will teach you how to just log informational steps which have no impact on the test outcome.
hello, i am just starting the selenium course and am having issues following along in section 2. i downloaded the code and wanted to run the test that dr. tiffiny showed in the ‘unit test’ section. however, i am getting error with ‘NuGet package.’ i’ve tried going to recommended page to restore NuGet, but my settings in VS are checked, as they say they should be. i am totally new to automation and coding and vs, so i am at a loss. can anyone help? here is a screenshot of the error message. thank you in advance!
https://uploads.disquscdn.com/images/de909bdd74168f418a365097deb6c85e829602c19e72019e10a3d5edb5f9d53e.png
On local scripts are working fine but while I try to execute on server, I am getting below error for firefox driver.
Root Cause: org.openqa.selenium.WebDriverException: newSession Build info: version: ‘3.11.0’, revision: ‘e59cfb3’, time: ‘2018-03-11T20:33:15.31Z’ System info: host: ‘USCKU1METY0119’, ip: ‘10.50.106.234’, os.name: ‘Windows Server 2012 R2’, os.arch: ‘amd64’, os.version: ‘6.3’, java.version: ‘1.8.0_191’ Driver info: driver.version: FirefoxDriver remote stacktrace: stack backtrace: 0: 0x4bb74f – 1: 0x4bbea9 – 2: 0x43ce8d – 3: 0x44ce14 – 4: 0x44944a – 5: 0x4203e1 – 6: 0x407dc7 – 7: 0x6d95b9 – 8: 0x4173a7 – 9: 0x6d38b3 – 10: 0x7ffb7e0d16ad – BaseThreadInitThunk
Im gotting issue while running ruby in windows
ERROR: Selenium::WebDriver::Remote::Capabilities does not have #dig method
One of the Selenium Ruby Devs will be able to help you here: https://seleniumhq.slack.com
sdfs
Hello,
I have an error like
“OpenQA.Selenium.NoSuchElementException: no such element: Unable to locate element: {“method”:”id”,”selector”:”userid”}
(Session info: chrome = 73.0.3683.86)
(Driver info: chromedriver = 2.41.578737)
at debug time running from cmd work fine at driver.FindElementById(“userid”)
but run time from chrome it will give error at this place.
I put everything like wait, wait till find element, full screen but still it’s not working when i run the process from web application.
It’s unable to locate the element that you’re searching for. The element with ID = userId . Probably a synchronization issue. Meaning that the element isn’t loaded when you search for it. Also, the element might be invisible and you might need to scroll to it to make it visible in the viewport
Thanks Nikolay, I tried with scrolling and visible viewport but still it will give me the same error.
I am so much confused that why it’s working properly when i run the process from webdriverscripting(Console application).
At that time driver find the userId properly but at same point it will give me the error from scheduler.
i am not sure but i guess it’s access issue may be i need to enable access to run the process.
It’s hard to say without seeing the actual HTML or the app 🙁
Thank you Nikolay for your support. As i said it’s issue from server access like in local system webdriverscripting having access but for the other user it’s not have access that’s why webdriverscripting not working properly. finally I did it. 🙂
Hi Nikolay,
I have asked a couple of questions on udemy course but seems like you haven’t responded to other queries as well. Hoping to get back reply from you.
Thanks,
Aditya
Hi Aditya,
What can I help you with? Been really busy
Hi Nikolay,
I am trying to automate a web page using C# and selenuim. I am trying to click on a widget in the GUI but it’s not working. I tried by giving the class name using ‘inspect element’ but it didn’t work. I saw that same class name has been given to other widgets too.
Received the below error when tried with class name:
{“invalid selector: Compound class names not permitted\n (Session info: chrome=74.0.3729.169)\n (Driver info: chromedriver=74.0.3729.6 (255758eccf3d244491b8a1317aa76e1ce10d57e9-refs/branch-heads/3729@{#29}),platform=Windows NT 10.0.15063 x86_64)”}
I am using Visual studio 2015 Enterprise edition
Please help me to resolve it.
Thanks,
RKrishnan
You can’t use compound locators like “xyz 123 space”, in that case you need to use Xpath
“Starting ChromeDriver (v2.10.267521) on port 22582 ” “Only local connections are allowed.”
Hello Nikolay, I just purchased the course and I am trying to get parallel for my Mac but the YouTube video link in the course doesn’t seem to be available anymore. I have seen a few videos on YouTube it I doubt their credibility. Please can you help?
George
Hello, Don’t worry about this anymore. I have bought a windows laptop.
Thanks
Parallels has excellent documentation, I recommend that you visit their website for instructions 🙂
Hello Nikolay, Please bear with me as I am completely new to automation. I have been following the video and I am stock on 185(How to download GitHub projects and open them). I down loaded your frame work and opened it in VS and had the following error:
“One or more projects in the solution were not loaded correctly. Please see Output window for details”
I selected OK and obviously saw a few more errors just as you did in the video. I did a build and realised that there were still some errors being thrown at me, please see below:
Severity Code Description Project File Line Suppression State
Error CS1513 } expected AutomationResources C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\WebDriverFactory.cs 39 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found CreatingReports C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\CreatingReports\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found ElementInteractions C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\ElementInteractions\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found LogginPractice C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\LogginPractice\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found SampleApp2 C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\SampleApp2\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found SauceLabs C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\SauceLabs\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found Selenium Grid C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\Selenium Grid\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS0006 Metadata file ‘C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\bin\Debug\AutomationResources.dll’ could not be found WebDriverTimeoutsTutorial C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\WebDriverTimeoutsTutorial\CSC 1 Active
Severity Code Description Project File Line Suppression State
Error CS1525 Invalid expression term ‘throw’ AutomationResources C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\WebDriverFactory.cs 39 Active
Severity Code Description Project File Line Suppression State
Error CS1002 ; expected AutomationResources C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\WebDriverFactory.cs 39 Active
Severity Code Description Project File Line Suppression State
Error CS1026 ) expected AutomationResources C:\Users\georg\Desktop\Kolade training plan\Automation with Udemy\LightPomFrameworkTutorial-master\AutomationResources\WebDriverFactory.cs 39 Active
Please any help will be very appreciated. As said before, I am completely and don’t know how to proceed.
Kind regards
Did you unzip the folder?
Hi guys I have this error the full stack trace:
Show raw exception details
OpenQA.Selenium.DriverServiceNotFoundException: The file C:\Inetpub\vhosts\pornagent.net\httpdocs\chromedriver.exe does not exist. The driver can be downloaded at http://chromedriver.storage.googleapis.com/index.html
at OpenQA.Selenium.DriverService..ctor(String servicePath, Int32 port, String driverServiceExecutableName, Uri driverServiceDownloadUrl)
at OpenQA.Selenium.Chrome.ChromeDriverService..ctor(String executablePath, String executableFileName, Int32 port)
at OpenQA.Selenium.Chrome.ChromeDriver..ctor(String chromeDriverDirectory, ChromeOptions options)
at coretest2.Controllers.HomeController.NewReleases() in C:\websites\coretest2\coretest2\Controllers\HomeController.cs:line 59
at lambda_method(Closure , Object , Object[] )
at Microsoft.Extensions.Internal.ObjectMethodExecutor.Execute(Object target, Object[] parameters)
at Microsoft.AspNetCore.Mvc.Internal.ActionMethodExecutor.SyncActionResultExecutor.Execute(IActionResultTypeMapper mapper, ObjectMethodExecutor executor, Object controller, Object[] arguments)
at Microsoft.AspNetCore.Mvc.Internal.ControllerActionInvoker.InvokeActionMethodAsync()
at Microsoft.AspNetCore.Mvc.Internal.ControllerActionInvoker.InvokeNextActionFilterAsync()
at Microsoft.AspNetCore.Mvc.Internal.ControllerActionInvoker.Rethrow(ActionExecutedContext context)
at Microsoft.AspNetCore.Mvc.Internal.ControllerActionInvoker.Next(State& next, Scope& scope, Object& state, Boolean& isCompleted)
at Microsoft.AspNetCore.Mvc.Internal.ControllerActionInvoker.InvokeInnerFilterAsync()
at Microsoft.AspNetCore.Mvc.Internal.ResourceInvoker.InvokeNextResourceFilter()
at Microsoft.AspNetCore.Mvc.Internal.ResourceInvoker.Rethrow(ResourceExecutedContext context)
at Microsoft.AspNetCore.Mvc.Internal.ResourceInvoker.Next(State& next, Scope& scope, Object& state, Boolean& isCompleted)
at Microsoft.AspNetCore.Mvc.Internal.ResourceInvoker.InvokeFilterPipelineAsync()
at Microsoft.AspNetCore.Mvc.Internal.ResourceInvoker.InvokeAsync()
at Microsoft.AspNetCore.Routing.EndpointMiddleware.Invoke(HttpContext httpContext)
at Microsoft.AspNetCore.Routing.EndpointRoutingMiddleware.Invoke(HttpContext httpContext)
at Microsoft.AspNetCore.Diagnostics.StatusCodePagesMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.Builder.Extensions.MapWhenMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.Session.SessionMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.Session.SessionMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.Authentication.AuthenticationMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.StaticFiles.StaticFileMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore.MigrationsEndPointMiddleware.Invoke(HttpContext context)
at Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore.DatabaseErrorPageMiddleware.Invoke(HttpContext httpContext)
at Microsoft.AspNetCore.Diagnostics.EntityFrameworkCore.DatabaseErrorPageMiddleware.Invoke(HttpContext httpContext)
at Microsoft.AspNetCore.Diagnostics.DeveloperExceptionPageMiddleware.Invoke(HttpContext context)
now on my local server is works perfectly but when I publish to my prodcution server the erorr appear.
some more specs I am doing asp.net core 2.2 mvc my server is server 2016 my browser chrome Version 76.0.3809.100
this are a few screen shots of my production server after I build the project
http://prntscr.com/oti0qn
http://prntscr.com/oti1gv
this is the code in Visual studio 2017
public ActionResult NewReleases()
{
string webRootPath = _hostingEnvironment.WebRootPath;
Guid g = Guid.NewGuid();
string filename = g + “.png”;
ChromeOptions options = new ChromeOptions();
options.AddArgument(“headless”);//Comment if we want to see the window.
//options.BinaryLocation = @”C:\Program Files (x86)\Google\Chrome\Application\chromedriver.exe”;
IWebDriver driver = new ChromeDriver(Path.GetDirectoryName(Assembly.GetExecutingAssembly().Location), options);
driver.Manage().Window.Size = new Size(1400, 800);
driver.Navigate().GoToUrl(“http://www.pornagent.net”);
var screenshot = (driver as ITakesScreenshot).GetScreenshot();
screenshot.SaveAsFile(string.Concat(webRootPath, “/SS/”, filename));
driver.Close();
driver.Quit();
const int size = 450;
const int quality = 80;
using (var image = new MagickImage(string.Concat(webRootPath, “/SS/”, filename)))
{
image.Resize(size, size);
image.Strip();
image.Quality = quality;
image.Write(string.Concat(webRootPath, “/SS/”, filename));
}
I was trying to solve this error for 3 days I am at my wits end I need help please
thanks in advance
Yeah I did. I have tried it again and am still getting the same errors. Thanks
HI, In Parallel execution (with [LoadTestRepeatCount()]),some times the buttons are not found and other elements are receive click action:”unknown error: Element … is not clickable at point (328, 150). ”
I use C# with selenium webdriver.
Any idea ..?
I have the solution under the title here: Element is not clickable at point (X, Y)
Hi Nikolay,
I completed your course and really learned a lot. You are a great teacher!
I am practicing with a sample project and built out a framework with a bunch of tests.
I am able to pass the tests when I run it as a single test in Chrome or Firefox, but if it is run all together in a group the tests are failing intermittently. I have flaky tests that are false failures if run together, but it passes when it runs by itself. Any advice on how to fix flaky tests? I’m not sure where to start in debugging this since I know the functionality is not broken and it is not a bug.
Can you provide some guidance?
I also have another question. Jobs ask for people to be able to build out a framework, but I feel I can only build out a simple framework but when it comes to complicated systems that requires complex reusable methods that involves databases for example I don’t have this knowledge or fear that I will struggle with coming up with the code to build out these complex reusable methods that requires very complex code. How does one move from the stage of being able to add/edit tests to be able to architect an entire framework?
Thanks.
Angee
Happy you were able to fix the webdriver wait.
Actually, the framework that you will learn to build here will be better than any framework you are likely to see in any job. There are only a handful of people in the world that can build a simple and clean framework such as the one that I teach you. I know this because I either work with or know most such people. Complexity in a framework != good. It’s bad and high maintenance. KISS and I promise you will be successful. If anyone tries to force you to be complex in your framework, talk to me 🙂
Hi Nikolay,
I am able to fix the intermittent failing flaky tests after adding in webdriverwait for all elements and fixing some locators to use something different. However, I notice it passes in Chrome, but fails in Firefox. I had to create a separate Firefox project and modify each element to wait for presence of element then it would pass on Firefox, but my original Chrome test is passing with each element to wait for visibility of element. Not sure why I can’t use one test for both browsers. How do you handle cross browser testing in one project with one test use for multiple browsers? If I put a lot of if this browser then use this element then each page object class will be over 200 lines which violates one of the principle you taught us in the automation class. Can you provide some guidance? I was wondering if you can code review my sample framework and provide any pointers on how to improve. How much do you charge to do a code review for my sample project? Can I purchase a code review session from you? Thanks for your help.
This is a very interesting problem for sure and it is really hard to know what’s going on here without seeing the code.
I think you didn’t get to Section 31 of the course yet. It will teach you how to keep POs small.
Yes, I do code and framework reviews all the time. Please reach out to me at nadvolod@gmail.com and we can discuss this.
Hi. I’m getting exactly this problem when running my tests using ChromeDriver and when using GeckoDriver: “Element is not clickable at point (X, Y). Other element would receive the click…” (Each browser says that the element is hidden by a different element.) Running the test suites via the Selenium browser extension works fine and it clicks on the element I’m trying to click on.
Your Solution seems to be incomplete for that problem, i.e. it says:
“You can scroll the element into view like this:
Or you can use the actions class to move to element before interacting with it like this:”
Please can you confirm the details of these 2 suggestions?
Thank you,
Sarah
Sorry, the code got lost. I updated the post with the code samples 🙂 Thanks so much for commenting and pointing that out
Thank you, Nikolay! I’ll try it out. 🙂
Maximizing the browser window before interacting with the element solved most of my “Element is not clickable at point (X, Y)” problems.
Thanks for the suggestion, I’ll add it to the solution!
After running a Selenium project on Chrome I switched to Safari and then back to Chrome. Now I get: “org.openqa.selenium.interactions.MoveTargetOutOfBoundsException: move target out of bounds” error for all tests in the project. Anybody have any ideas?
For the first error “There is already an option for the browserVersion capability. Please use the instead.
Error Message” , “Here’s a code sample for C#:” , but I did not find the c# sample code, could you please add, thanks!
For the first error “There is already an option for the browserVersion capability. Please use the instead.” , I did not find C# sample code, could you please add? Thanks.
Fixed
I have tried to open few forms using selenium driver. For 2 pages I am able to navigate .But when onn 2nd form I am trying to click on next button.3 rd page is showing loading only and its not loaded properly. Wherever When I am taking the same URL of 3rd page to new manualay opened window of chrome ,Its opening…Any suggestions?
Any Suggestions ….?
I don’t 100% understand your question. Can you please explain step by step what you are doing and what you are hoping to accomplish? Thanks
I usually gets Null Reference Exception.. How can i solve this?
For example while taking the testName from TestContext and assigning to a new string am getting this error.
Below are my codes
public TestContext testContext { get; set;}
public string testname;
public TestIntialize() {
testname=testContext.TestName;
}
Here i getting error saying null value is referenced by TestName
How can i solve this
NullReference means that the object isn’t initialized when you try to use it. Turn you your debugger, step though the code and see which element is null when you try to use it. Based on your code, I will guess that your `testContext` is null when it ties to do `.TestName`
Hi, I tried to run some test of LightPomFramework, always I got an issue try to solve it and then the test passed. But now I’m tired to doing that… because I can’t progress with the course.
The output is
SeleniumElementLocationExam
Duration: 13 sec
Message:
Test method ElementInteractions.IdentifyingWebElements.SeleniumElementLocationExam threw exception:
OpenQA.Selenium.WebDriverException: unknown error: Element is not clickable at point (112, 668). Other element would receive the click: …
(Session info: chrome=80.0.3987.149)
(Driver info: chromedriver=2.37.544315 (730aa6a5fdba159ac9f4c1e8cbc59bf1b5ce12b7),platform=Windows NT 10.0.18363 x86_64)
Stack Trace:
RemoteWebDriver.UnpackAndThrowOnError(Response errorResponse)
RemoteWebDriver.Execute(String driverCommandToExecute, Dictionary`2 parameters)
RemoteWebElement.Execute(String commandToExecute, Dictionary`2 parameters)
RemoteWebElement.Click()
IdentifyingWebElements.SeleniumElementLocationExam() line 93
Please, someone can help me?
Please see error 2.20 here please https://ultimateqa.com/common-selenium-webdriver-errors-fix/
Hi Nikolay,
I implement the suggested solution on https://ultimateqa.com/common-selenium-webdriver-errors-fix/#Element_is_not_clickable_at_point_X_Y_Other_element_would_receive_the_click
using
private void MoveToElement(By locationStrategy)
{
var js = (IJavaScriptExecutor)Driver;
js.ExecuteScript(“arguments[0].scrollIntoView({behavior: ‘smooth’, block: ‘center’})”, locationStrategy);
}
But It’s not working.
Hi Nikolay,
I want to know how i can handle Untrusted SSL certificate error in IE browser through C#. I have seen if its Chrome, I can use ChromeOptions, but how to resolve if its IE in C#?
Hi,
I want to how to convert Selenium C# project to a executable similar to jar files in java, to be executed in some other machines, without actually exposing my code.
Hello. I am taking the course Selenium WebDriver Master Class with C#. When I go to 03 Programming Basics, I run in debug mode the file Program.cs under HelloWorld. It gives me errors like “CS0169 – The field Car.Speed is never used. CS0162 Unreachable code detected.
This is what is in the file. I do not change anything. As a matter of fact, any cs file I run generates errors.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Hello.World
{
class Program
{
static void Main(string[] args)
{
// The code provided will print ‘Hello World’ to the console.
// Press Ctrl+F5 (or go to Debug > Start Without Debugging) to run your app.
//Console.WriteLine(“Hello World!”);
//Console.ReadKey();
// Go to http://aka.ms/dotnet-get-started-console to continue learning how to build a console app!
var table = string.Format(“{0, 10} {1,10}”, “test”, “2”);
var dollarFormat = string.Format(“{0:C}”,2);
Console.WriteLine(table);
Console.WriteLine(dollarFormat);
}
}
}
Hello. I am not sure if my comment was accepted, but I have downloaded the github solution for “Selenium WebDriver Masterclass C#”. Whenever I run any class file, ex. Program.cs, it is always failing, even though I have not made any changes after downloading from GitHub. There seems to be something wrong in Section 6, but I am running the first class file in Section 3.
Ex. Error: CS0169 – The field ‘Car.speed’ is never used (File Car.cs). Error: CS0162: Unreachable code detected (File ForLoops.cs).
Hi Mark, what is your version of Visual Studio and .NET that you are using?
Hi. VS2019 and it shows .NET Framework 4.6.1 when I right click on the Section 4 Properties. Thanks.
Mark
Mark, Are you referring to this? https://www.evernote.com/l/AWSk4VUhqMZFwqxtcj3D7je_F7pX2YKgXJI
Yes, it is.
Those are warnings 🙂 Not blocking errors. You are free to proceed with no problems
Thank you for your comments. Mark
hey tony have you found a solution due the regarding comment? I have the very same issue here.
I had the same error
You mean the Unreachable code detected? As Nikolai mentioned, this is only a warning and you should be able to continue working on the project without any issue.
Hi Nikolay,
Selenium C# – would you advise to use the WebDriverManager instead of downloading and configuring each browser driver?
Thanks,
Mike
Hi Mike,
If you use WebDriverManager, then it will keep your drivers up-to-date with less work from your part, so I would say go for it
Andreea
Many thanks for your reply Andreea.
Hi ,
im unable to launch IE browser getting below issue even though enabling the protected mode.
Test method TestProject2.UnitTest1.TestIE threw exception:
System.InvalidOperationException: Unexpected error launching Internet Explorer. Protected Mode settings are not the same for all zones. Enable Protected Mode must be set to the same value (enabled or disabled) for all zones. (SessionNotCreated)
Hi Rakesh!
This seems to be related to your browser settings. edit Internet Options on your computer and enable Protected mode in all zones – Internet, Local internet. Trusted sites, and Restricted sites. This should solve your problem.
Nikolay Advolodkin ,
I’m getting below error , i’m not understanding whats the issue, i’m using eclipse IDE 2020-09 with jDK compliance 1.7
Could you please help me on below issue
Thank in advance 🙂
**Console error**
Picked up _JAVA_OPTIONS: -Xmx1024M
Exception in thread “main” java.lang.ExceptionInInitializerError
at org.apache.poi.ss.util.CellReference.(CellReference.java:110)
at org.apache.poi.xssf.usermodel.XSSFCell.(XSSFCell.java:119)
at org.apache.poi.xssf.usermodel.XSSFRow.(XSSFRow.java:77)
at org.apache.poi.xssf.usermodel.XSSFSheet.initRows(XSSFSheet.java:268)
at org.apache.poi.xssf.usermodel.XSSFSheet.read(XSSFSheet.java:231)
at org.apache.poi.xssf.usermodel.XSSFSheet.onDocumentRead(XSSFSheet.java:218)
at org.apache.poi.xssf.usermodel.XSSFWorkbook.parseSheet(XSSFWorkbook.java:454)
at org.apache.poi.xssf.usermodel.XSSFWorkbook.onDocumentRead(XSSFWorkbook.java:419)
at org.apache.poi.ooxml.POIXMLDocument.load(POIXMLDocument.java:184)
at org.apache.poi.xssf.usermodel.XSSFWorkbook.(XSSFWorkbook.java:288)
at org.apache.poi.xssf.usermodel.XSSFWorkbook.(XSSFWorkbook.java:309)
at com.test.utilities.XLUtils.main(XLUtils.java:16)
Caused by: java.nio.charset.UnsupportedCharsetException: Big5
at java.base/java.nio.charset.Charset.forName(Unknown Source)
at org.apache.poi.util.StringUtil.(StringUtil.java:39)
12 more
while executing the below selenium code
public class XLUtils{
public static void main(String[] args) throws Exception
{
FileInputStream file = new FileInputStream(“C:\Selenium\LoginData.xlsx”);
XSSFWorkbook workbook = new XSSFWorkbook(file);
XSSFSheet sheet=workbook.getSheet(“Sheet1”);
int rowcount=sheet.getLastRowNum();
int colcount=sheet.getRow(0).getLastCellNum();
for(int i=1;i<rowcount;i++)
{XSSFRow currentrow = sheet.getRow(i);
for(int j=0;j<colcount;j++)
{String value =currentrow.getCell(j).toString();
System.out.print(value);
}System.out.println();
}
}
}
Hi, unfortunately I don’t know Eclipse
Was getting errors re chromedriver being out of date on the Test2_ImplicitWaitExample(), so I tried to put in a newer chromedriver.exe version where it said the error was, no luck. So I downloaded the whole solution again, tried to build, and get the following error. I don’t know how to fix this. And I don’t know how to fix the implicitwaitexample test case when I get to that again.
Severity Code Description Project File Line Suppression State
Error Could not copy the file “C:\Users\BEgbers\source\repos\LightPomFrameworkTutorial-master (2)\LightPomFrameworkTutorial-master\packages\WebDriver.ChromeDriver.win32.85.0.4183.87\content\chromedriver.exe” because it was not found. LogginPractice
Hi Beth,
Before you try to update it again, can you please check that no chromedriver.exe processes are still running? You can do that from Task Manager. Then, make sure that you have a chromedriver version that matches your Chrome browser version and copy it again.
Hi, Thanks for responding, That issue got resolved and its due to old version of library file which has now replaced with stable version of files and the error reported above is no longer exists.
Hi Nicolay, I face onetimesetup: system.Net.WebException error while using webdrivermanager to launch firefox browser while the same work for Chrome browser.
Hi Nidhi,
Can you take a look at the answer on this post and see if you can solve your problem the same way? https://stackoverflow.com/questions/13276686/selenium-webdriver-tests-fail-with-system-net-webexception-unable-to-connect
Hi Nikolay, Is there any step by step to install Selenium in windows 10? I was waiting the installation of selenium in the course. Thanks.
jpatino2580@gmail.com
Hi Julian!
You don’t need to do anything different on Windows 10, the steps to add Selenium to your projects are the same.
This article is exceptionally valuable and informative. Much appreciated
Helpfu. Thanks for writing. Will execute it the next time.
How is stating there is a bug, a solution?
Hello Nikolai, i am on section 19, video 207(Highlighting all web elements using Selenium) and i have a problem that using AutomationResources could not be found, even if i typed that on the first line, so the WebDriverFactory, BrowserType, HighlightElementUsingJavaScript wont work at all.
Thanks