I think that you will agree with me when I say that in the history of test automation, Selenium and Appium are the two of the greatest tools that have been created. They are the de facto standard for mobile and web automation.
Unfortunately, they are only the bare bones that are required to actually do test automation at the workplace. Along with downloading Selenium and Appium, you will also need to pick a test runner, a reporting tool, a logging tool, and potentially others.
However, there are amazing tools that exist that make it drastically easier to get started with test automation. These tools make setup, maintenance, and usage of test automation magnitudes simpler.
Stay with me as you learn how to level up your test automation.
TestProject
I learned about TestProject.io about a year ago and I was so impressed that I decided to try it out.
TestProject is a free end-to-end test automation platform for web, mobile, and API testing that’s supported by the #1 test automation community.
https://testproject.io/
In short, TestProject will help you to decrease software maintenance costs and decrease startup costs.
Let’s dive in and find out why you need to experiment with this tool.
Built on Open Source
Similar to all the other tools in this post, TestProject is built using at least Selenium and Appium. This automatically means that you have a single tool to do both mobile and web automation.
TestProject works through agents that you download to your machine. The agents basically wrap Selenium and Appium into the latest and greatest working software, along with any necessary drivers.
Hence, since you no longer need to download a bunch of packages and drivers, these agents drastically reduce your maintenance time. Allowing you to get up and running with your test automation even faster.
Test #automation is really hard! As we're working through the process, it's always good to be mindful of the decisions that you are making. Always ask yourself, "Am I increasing complexity and as a result maintenance costs"? If so, it's most… Share on XOnce you install the agent, you can now easily use the TestProject web UI to interact with your web or mobile application to automate it. It will look something like this:

Community Developed Addons
A plugin architecture that allows for the community to extend the behavior of the software is always an amazing idea! TestProject makes this possible by allowing the community to develop addons that can then be utilized by all the users.
You can also create your own addons that you can share with your team or with the entire TestProject community if you wish. Addons make it easy to customize the actions you have in your tests to exactly fit your team’s particular testing needs.
https://docs.testproject.io/testproject-addons/what-is-an-addon
Here are just a few of the examples of the type of addons that can exist and what they can help with:

Check out all of the add ons here.
After you install the addons that you want, simply use them in your test recordings by picking the appropriate actions.

Obviously, the power of addons is that TestProject functionality is extendable by the community.
It gets even better:
You have the capability to use add-ons in your coded tests as well. Currently, TestProject supports both Java and C# SKDs for your coded tests.
So your tests might look something like this:
If you’re looking for more code samples, TestProject has a repo of C# SDK examples and Java SDK examples.
Why TestProject?
In summary, go grab your free copy of TestProject.io and get ready to decrease maintenance and startup costs. First, the drivers and frameworks are automatically maintained when you download the agent. That means mobile and web automation from a single download! 👏
Second, if you need extra functionality from the tool, you can develop your own addon, or get one from the community. And we all know how two brains are always better than one.
Finally, it’s absolutely free 👏👏 So you have nothing to lose, except your stress 😂
PS. If you want to get started quickly here’s a video tutorial on how to get started in under 20 minutes.
Webdriver IO
This year I was introduced to one of the best test automation tools that I have ever used by the name of Webdriver IO. If you have not heard about this tool, pay attention, it’s excellent.
Webdriver IO is a Node.js automation framework that uses WebDriver W3C protocol for web and mobile automation under the hood. What makes it really awesome is the easy setup and the simple interface that exists to interact with your applications.
Easy Setup of the Tool
The wdio command line interface comes with a nice configuration utility that helps you to create your config file in less than a minute. It also gives an overview of all available 3rd party packages like framework adaptions, reporter and services and installs them for you.
https://webdriver.io/
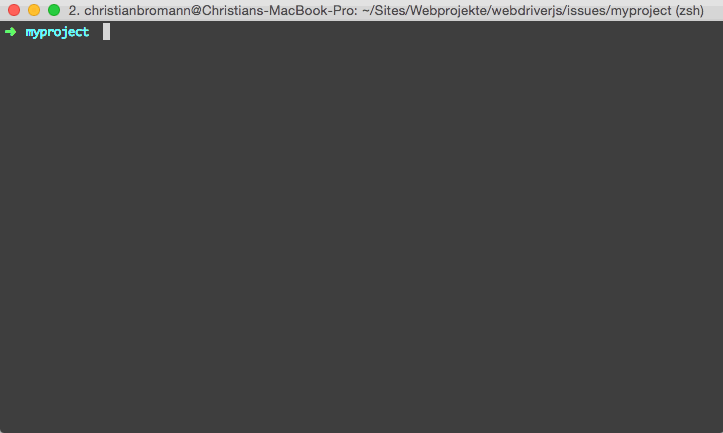
It’s the best setup of an automation framework that I have seen to date. And I have been around and seen other frameworks that exist in .NET and Java.
It gets better:
I’m further really impressed by the fact that through the command line you can install almost any dependency you need to have a full automation framework. Reporting tools, unit testing tools, and connect to services like Sauce Labs. All by answering a few of the questions that the installer asks you.
At the end of a few minutes, you’re ready to start coding your automation, fully integrated into all the frameworks that you want.
Well Thought Out API
I think that you will agree with me when I say that the API of Selenium or Appium is not the most user-friendly. But this is fine because these are the interfaces that are designed for us to interact with the software. That’s where excellent tools like Webdriver IO come into play.
The Webdriver IO does an excellent job of wrapping Selenium and Appium into an API that we can use to interact with our applications. Take a look:
//Sample HTML
<button id="myButton" onclick="document.getElementById('someText').innerHTML='I was clicked'">Click me</button>
<div id="someText">I was not clicked</div>
//Click a button
const myButton = $('#myButton');
myButton.click();
The equivalent with Selenium would look something like this:
var myButton = driver.FindElement(By.Id("myButton"));
myButton.click();
The old approach isn’t horrible, but it is more verbose. Doing this all throughout our automation code can certainly take extra time and effort.
Why Webdriver IO?
Whether or not you are using Node.JS, everyone needs to take a look at this framework. It’s an excellent wrapper around the Selenium and Appium APIs. As a result, the process of setting up your framework to start coding automation is magnitudes simpler. Furthermore, the user-friendly API further decreases the complexity for us to do simple actions against our software under test.
Watir
Watir is an excellent Ruby library for automating web applications and working on adding mobile support through Appium as well.
The main reason that this library is on this list is that it has a really advanced API that allows the automation engineer to write cleaner and more robust automation code.
Let’s take a look:
Watir uses advanced waiting strategy
As you may already know, Selenium has an implicit and an explicit wait that you can use to make sure that the element is in a correct state, before interacting with it. However, the recommended approach is to only use explicit waits.
Rather than forcing you to understand the mechanics, Watir has taken that load off your shoulders and has a built-in waiting strategy to reduce test flakiness.
This is how most of us interact with an element today:
browser.text_field(title: 'Search').when_present.set 'Hello World!'
The problem here is that you should never be using #set
if you aren’t sure the element will be there or enabled, so these #when_present
calls are philosophically redundant.
As such, Watir 6.0 deprecated
http://watir.com/guides/waiting/#when_present
and#when_enabled
and every action call on an element effectively executes this logic by default.
Note that Watir does its automatic waiting when taking actions, not when attempting to locate. This provides additional flexibility for querying the state of an element without needing unnecessary waits.
Nice, right?
It’s something that all test automation frameworks should implement, regardless of the programming language.
Watir does advanced element location
Using element locators with Selenium can be a bit tricky and many of us have been known to come up with complicated locators to find that one unique element that we want to interact with.
Watir takes away some of that complexity by providing more locator options.
You can provide an Array of String or RegExp values like this:
browser.text_field(class: ['order', 'should', 'matter', 'not'])
browser.text_field(class: ['this', '!notthis'])
Which is super handy if you want to narrow down your collection of elements.
Even better, you can find adjacent nodes without having to come up with funky CSS syntax. Like this:
anchor_element.parent(selectors)
anchor_element.previous_sibling(selectors)
anchor_element.following_sibling(selectors)
anchor_element.siblings(selectors)
anchor_element.child(selectors)
anchor_element.children(selectors)
Why Watir?
As you can tell, Watir is just a really friendly wrapper around the WebDriver protocol. It takes what is already good about WebDriver and enhances certain aspects to make test automation easier and more robust. Exactly what a good automation tool should do.
Conclusions
TestProject.io, Webdriver IO, and Watir are some tools that you must try today. They make Selenium and Appium drastically easier to use and will push your automation to the next level.
Have you tried any of these tools and what did you think? 🤔
Trackbacks/Pingbacks